- $.globalEval in native javascript 29 July 2013
- Back button issue with Iframes 30 June 2013
- Issue with onbeforeunload on IE 26 June 2013
- text truncation using css 18 June 2013
- Date issue in ie8 28 May 2013
- postMessage API 23 May 2013
- Issue with mutating page title with jQuery 17 May 2013
- Dreaded 31 style/link tags limit in IE 8/9 10 May 2013
- Overriding backbone's event hash 06 May 2013
- Crossbrowser image rotation 03 May 2013
- Access events of a DOM node jQuery 20 April 2013
- Load js/css without parsing and execution 26 March 2013
- meta charset='utf 8' what the fuss? 20 March 2013
- Web Storage 19 March 2013
- setTimeout(function(){},0) 19 March 2013
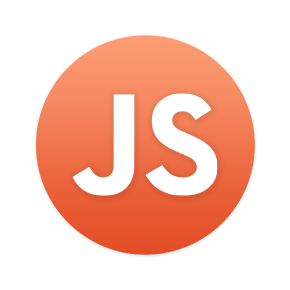
eval
allows developers to execute code and create variables in the scope in which it is executed.
For Example:
function evalExec(){ eval('var a=10;'); } evalExec();
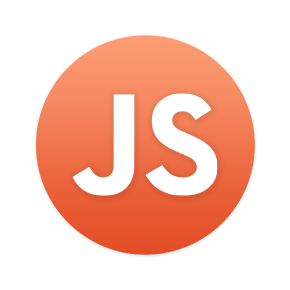
Ever stuck with a problem/issue with browser back button
and an iFrame
on a webpage? If yes, read on.
Consider a scenerio where we have an iframe inside a webpage. When user clicks any links present inside iframe the iframe's content changes and it displays a new page.
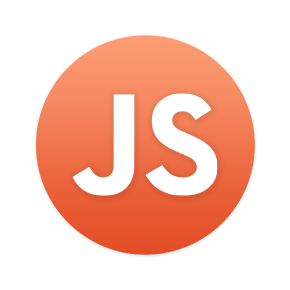
What is onbeforeunload?
Accourding to MDN docs its an event which fires when a page is about to be unloaded. This event is cancelable and is presented as a modal box to the user. Here is the image of the modal box shown by jsfiddle.net when someone tries to navigate away.
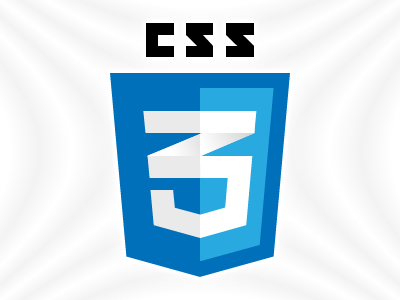
Ever wondered how to truncate text using only css with no javascript and apply ellipsis (...) at the end of the text.
Here is the solution.
.ellipse{ width:240px; overflow:hidden; white-space:nowrap; text-overflow:ellipsis; }
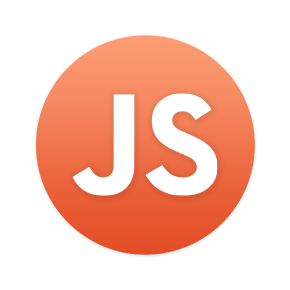
I was left stunned when I encountered this wierd IE8 issue. My flow involved taking input from the user via a calender and convert that input into date. I used a datepicker which returned me date string in the format yyyy-mm-dd
.
I took the values selected by user and pass it ot the Date constructor in javascirpt and it worked in FF, Chrome, IE8+. But as soon as i tried the same in IE8 it Bombed
and left me wondering why?
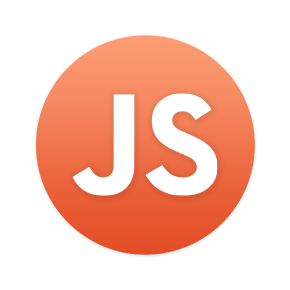
Think of cross window, cross iframe communication and what comes to mind is postMessage
API. This postMessage api allows windows(iframes) to communicate among themselves.
One window can post a message to other message and the other can react to the message upon recieving it. Lets see how it works.
Code in Message Sender window
var window2=window.open("/someurl.html"); /* This url can be anything but it should point to page which is the reciever */ window2.postMessage("Hello window 2","http://devangpaliwal.com");
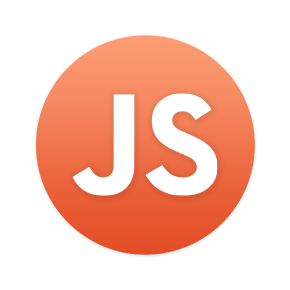
If we have to change an element's content using jQuery we can use the html()
or text()
functions
provided by the library. This works like a charm until we are not dealing with title element in Internet Explorer
.
Yeah, I was surprised to see IE throwing error when asked to changed the title using jQuery's html() method. My code
$("title").html("Js Blog");
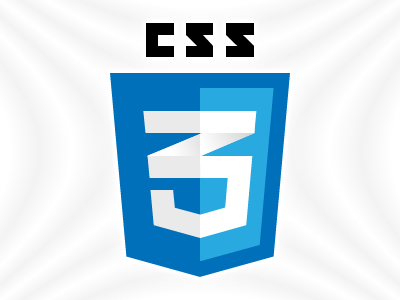
I lost lot of hairs while debugging a rich webpage in IE 9, by rich i mean having lot of js and css. In development
mode, the scripts and css are not minified and combined into a single js, css file. Yeah, so my page had around 35 to 40 links and style tags.
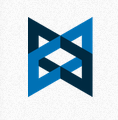
Backbone provides a declarative way of binding events to the respective dom of a view object. For example:
events:{ ".close click":"closeMe", ".delete click":"deleteMe" }
All events listed in the event's hash will be attached to the dom and corresponding function will be called when listed event happens on selected elements.
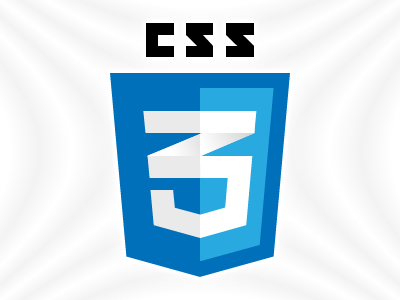
Image rotation is a key requirement that any front-end guy encounters while doing a web project. For example:
Lets say we have a slideshow which can be configured to work both horizontally
as well as vertically
. For navigation we have an image of a arrow pointing to the right.
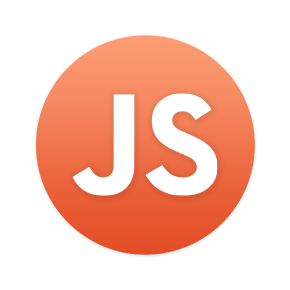
Ever wondered how and where jQuery stores event handlers for a dom node
and how we can access all the event handlers ?
jQuery stores event handlers for a dom node in the events store. It can be accesses via the data
method of the dom node.
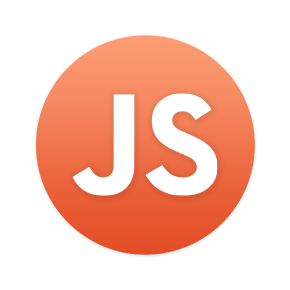
Usecase: Lets say we have a workflow on our website which follows a particular path, for example after signup we ask user to complete his user profile. In order to make the complete profile page experience faster we can download the required resources of the profile page at signup page itself.
Note that i said just download, not parse and execute
. By doing this we download all the javascripts,css and images of the profile page while the user is filling up the registration details.
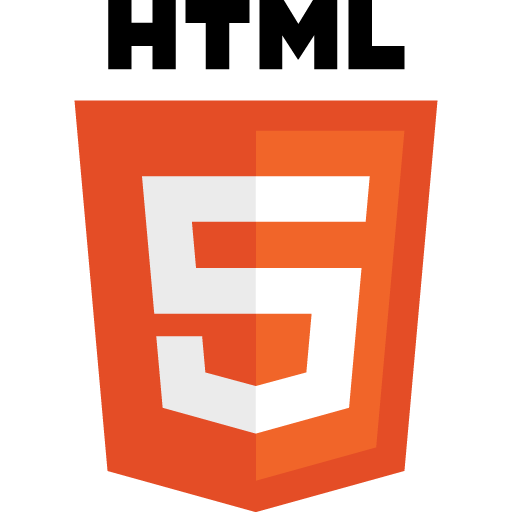
I have seen html developers writing below piece of code in the head tag of the html document.
<meta charset='utf-8'> OR <meta http-equiv='Content-Type' content='text/html; charset=utf-8'>
What does charset='utf-8'
mean? What if this tag is ommited from the document?
Charset is used to specify character encoding.
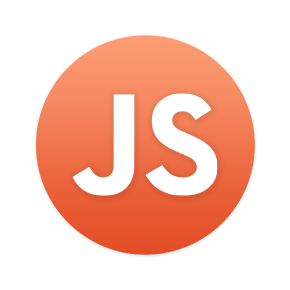
W3C has defined a webstorage spec which deals with the task of storing data on the web clients. Prior to this spec, developers used to store key/value pairs in cookies.
Cookies are good but they have few drawbacks like the maximum amount of data(4kb) per domain and all cookies are sent to server along with each request adding to request overhead.
Webstorage solves this problem by allowing storage size of minimum 5mb per domain, also the data stored in the storage is not passed to the server with every request.
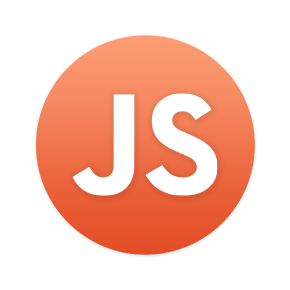
Sometimes i needed to do it in my code. I wanted to understand how did this solved my issue and why?:
var callMeLater=function(){ } setTimeout(callMeLater,0);
Browser is responsible for so many thigs like loading files, interpreting html, rendering html, executing javascript. Browser uses multithreading to do its tasks in parallel. One thread handles rendering of html, other takes care of downloading js,css and other files.